Version control is an essential skill for developers and anyone involved in managing code or documents. Git, a distributed version control system, and GitHub, a popular platform for hosting Git repositories, are powerful tools that help track changes, collaborate with others, and maintain code quality. This guide will introduce you to Git and GitHub, covering basic commands, branching, merging, and collaborating effectively.
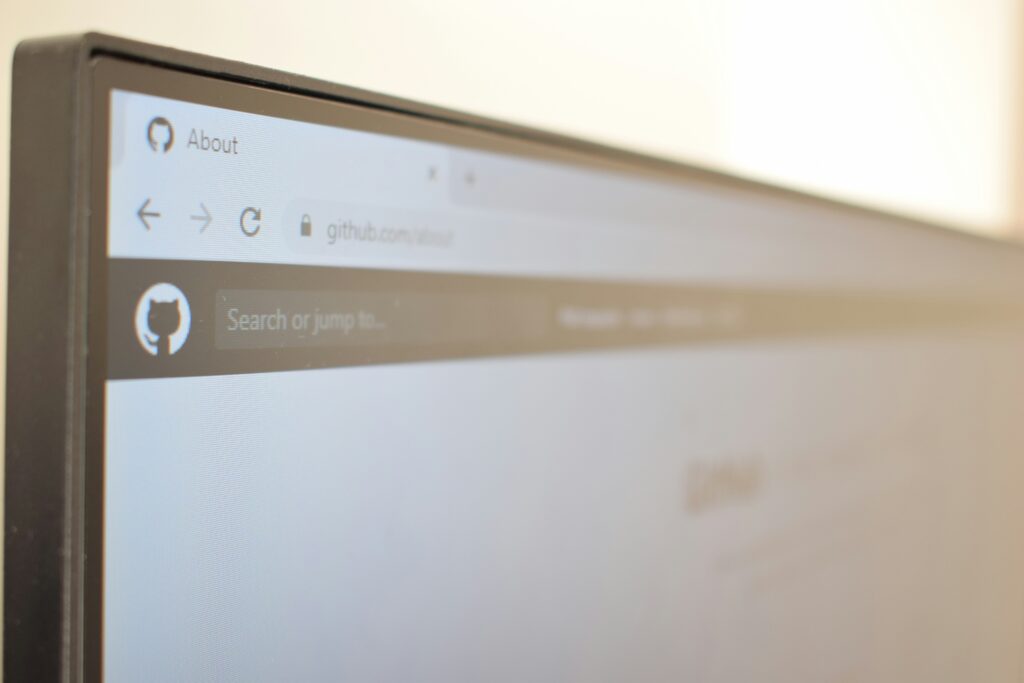
What is Git and GitHub?
- Git: A version control system that allows you to track changes to your files and collaborate with others. Git manages changes in your codebase and helps you revert to previous versions if necessary.
- GitHub: A cloud-based platform that hosts Git repositories, providing additional tools for collaboration, code review, and project management.
Step 1: Installing Git
- Download Git: Visit the official Git website and download the installer for your operating system.
- Install Git: Follow the installation instructions. On Windows, you can use the default settings or customize as needed. On macOS and Linux, you can use package managers like Homebrew or apt-get.
- Verify Installation: Open your terminal or command prompt and run:
git --version
This command will display the installed Git version.
Step 2: Configuring Git
Before using Git, set up your name and email, which will be used in your commit messages:
- Set User Information:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
The--global
flag sets these details for all repositories on your system. You can override these settings for individual repositories if needed. - Check Configuration:
git config --list
This command lists all your Git settings.
Step 3: Creating a Git Repository
- Initialize a Repository: Navigate to your project directory and run:
git init
This command creates a new Git repository in the directory. - Clone an Existing Repository: If you want to contribute to an existing project, you can clone it:
git clone https://github.com/username/repository.git
Replaceusername/repository.git
with the URL of the GitHub repository.
Step 4: Basic Git Commands
- Check Repository Status:
git status
This command shows the status of changes in your working directory and staging area. - Add Changes: To stage changes for commit:
git add filename
Usegit add .
to add all changed files. - Commit Changes: Save your changes with a commit message:
git commit -m "Your commit message"
- View Commit History: To view the commit history:
git log
- Undo Changes:
- To unstage a file:
git reset filename
- To discard changes in a file:
git checkout -- filename
- To unstage a file:
Step 5: Branching and Merging
Branches allow you to work on different features or fixes independently:
- Create a New Branch:
git branch branch-name
Replacebranch-name
with your desired branch name. - Switch to a Branch:
git checkout branch-name
- Create and Switch to a Branch in One Command:
git checkout -b branch-name
- Merge Branches: First, switch to the branch you want to merge into (typically
main
ormaster
):git checkout main
Then merge the desired branch:git merge branch-name
- Resolve Merge Conflicts: If there are conflicts, Git will prompt you to resolve them. Open the conflicted files, make the necessary changes, and then:
git add filename git commit
Step 6: Collaborating with GitHub
- Create a GitHub Account: Sign up for a free GitHub account at github.com.
- Create a New Repository:
- Go to your GitHub profile.
- Click on the “Repositories” tab and then “New” to create a new repository.
- Follow the prompts to name and initialize your repository.
- Push Local Changes to GitHub:
- Add a remote repository:
git remote add origin https://github.com/username/repository.git
Push your changes:
git push -u origin main
-u
flag sets the upstream branch for themain
branch. - Add a remote repository:
- Pull Changes from GitHub: To update your local repository with changes from GitHub:
git pull origin main
- Create and Manage Pull Requests:
- Push your branch to GitHub:
git push origin branch-name
- Go to your repository on GitHub, switch to your branch, and click “New pull request.”
- Review the changes, add comments, and submit the pull request.
- Push your branch to GitHub:
Step 7: Best Practices
- Commit Often: Make regular commits to save your progress and maintain a clear history of changes.
- Write Clear Commit Messages: Use descriptive commit messages to explain what changes were made and why.
- Use Branches for Features: Create separate branches for new features, bug fixes, or experiments to keep your main branch stable.
- Review Pull Requests: When collaborating, review pull requests thoroughly to ensure code quality and consistency.
- Keep Your Repository Organized: Use
.gitignore
to exclude unnecessary files and keep your repository clean.
Conclusion
Git and GitHub are powerful tools for version control and collaboration. By mastering basic Git commands, understanding branching and merging, and utilizing GitHub for collaboration, you’ll be well-equipped to manage your projects effectively. As you gain more experience, you can explore advanced features and workflows to further enhance your version control skills.
Feel free to ask if you have any questions or need more detailed explanations on any specific Git or GitHub feature!