Machine learning (ML) is revolutionizing industries by enabling computers to learn from data and make predictions or decisions without explicit programming. Whether you’re interested in data science, artificial intelligence, or just curious about machine learning, Python offers an excellent starting point due to its simplicity, powerful libraries, and vast community support.
This step-by-step tutorial will introduce you to the basics of machine learning using Python. We will cover setting up your environment, essential Python libraries for ML, and build a simple ML model to predict house prices.
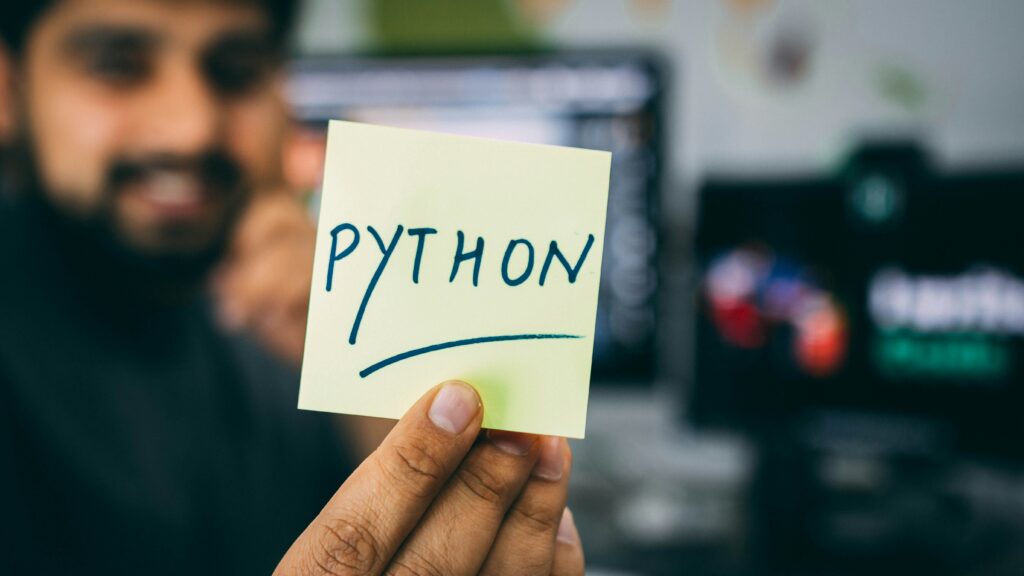
Step 1: Setting Up Your Python Environment
Before diving into machine learning, you need to set up your Python environment. Here’s what you need:
- Install Python: If you haven’t already, download and install the latest version of Python from the official website. Ensure that you add Python to your system path during installation.
- Install Anaconda (Recommended): Anaconda is a popular distribution for Python and data science. It comes pre-packaged with most ML libraries and tools. Download and install Anaconda from the official website.
- Set Up Jupyter Notebook: Jupyter Notebook is an open-source web application that allows you to create and share documents containing live code, equations, visualizations, and text. It’s ideal for experimenting with machine learning models. To install Jupyter Notebook, open your terminal or command prompt and run:
codepip install jupyter
Launch Jupyter Notebook by typing:
codejupyter notebook
Step 2: Install Essential Python Libraries
To get started with machine learning, you will need the following libraries:
- NumPy: A fundamental package for numerical computing.
- Pandas: A powerful data manipulation and analysis library.
- Matplotlib and Seaborn: Libraries for data visualization.
- Scikit-Learn: A popular ML library that provides simple and efficient tools for data mining and data analysis.
Install these libraries by running:
pip install numpy pandas matplotlib seaborn scikit-learn
Step 3: Understanding the Basics of Machine Learning
Machine learning involves teaching computers to learn patterns from data to make predictions or decisions. There are three main types of machine learning:
- Supervised Learning: The model is trained on labelled data. For example, predicting house prices based on historical data.
- Unsupervised Learning: The model identifies patterns in unlabeled data, such as grouping customers into different segments.
- Reinforcement Learning: The model learns by interacting with its environment and receiving feedback in the form of rewards or penalties.
In this tutorial, we’ll focus on Supervised Learning, where our goal is to train a model to predict a continuous value (regression problem) using labelled data.
Step 4: Loading and Understanding the Dataset
We’ll use the popular Boston Housing dataset, which contains information about various factors affecting house prices in Boston.
- Import Required Libraries:
import numpy as np import pandas as pd import matplotlib.pyplot as plt import seaborn as sns from sklearn.datasets import load_boston from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error
- Load the Dataset:
# Load the Boston Housing dataset boston = load_boston() # Convert to DataFrame for easy manipulation data = pd.DataFrame(boston.data, columns=boston.feature_names) data['PRICE'] = boston.target data.head()
The dataset includes features like the number of rooms, crime rate, property tax rate, and the target variable (house prices). - Explore the Dataset:
# Check for missing values print(data.isnull().sum()) # Display dataset statistics print(data.describe()) # Visualize the distribution of the target variable (house prices) sns.histplot(data['PRICE'], bins=30, kde=True) plt.show()
Step 5: Visualize Relationships in the Data
Visualizing the relationships between different features can help you understand the data better and identify patterns:
# Visualize the correlation matrix
plt.figure(figsize=(10, 8))
sns.heatmap(data.corr(), annot=True, cmap='coolwarm')
plt.show()
This heatmap shows the correlation between different features and the target variable, PRICE
. Strong correlations can indicate important predictors.
Step 6: Preparing the Data for Training
Before training the model, we need to prepare our data:
- Select Features and Target Variable:
X = data.drop('PRICE', axis=1) # Features y = data['PRICE'] # Target variable
- Split the Data into Training and Testing Sets:
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Splitting the data ensures that the model is trained on one subset and tested on another to evaluate its performance.
Step 7: Building a Simple Machine Learning Model
Now, we’ll build a simple linear regression model using Scikit-Learn:
- Initialize and Train the Model:
model = LinearRegression() model.fit(X_train, y_train)
- Make Predictions:
y_pred = model.predict(X_test)
- Evaluate the Model:
# Calculate Mean Squared Error (MSE) mse = mean_squared_error(y_test, y_pred) print(f"Mean Squared Error: {mse:.2f}") # Visualize the Actual vs. Predicted Prices plt.scatter(y_test, y_pred) plt.xlabel('Actual Prices') plt.ylabel('Predicted Prices') plt.title('Actual vs. Predicted Prices') plt.show()
Step 8: Interpreting the Results
- Mean Squared Error (MSE) measures the average squared difference between the predicted and actual values. A lower MSE indicates a better fit.
- The scatter plot of actual vs. predicted prices helps visualize the model’s performance. If the points closely align along the diagonal, the model performs well.
Step 9: Improving the Model
To improve the model’s performance, consider the following techniques:
- Feature Scaling: Standardize or normalize features to improve model convergence and performance.
- Feature Engineering: Create new features from existing ones to provide more meaningful information to the model.
- Hyperparameter Tuning: Adjust model parameters, such as the learning rate, to optimize performance.
- Cross-Validation: Use techniques like k-fold cross-validation to evaluate the model’s performance more robustly.
Step 10: Next Steps in Machine Learning
Congratulations! You’ve built your first machine learning model in Python. Here are some next steps to continue your learning journey:
- Explore More Algorithms: Experiment with different ML algorithms, such as decision trees, support vector machines, and neural networks.
- Dive Deeper into Data Preprocessing: Learn more about data cleaning, handling missing values, and feature selection.
- Advanced Topics: Explore advanced ML topics like deep learning, natural language processing (NLP), and reinforcement learning.
Conclusion
Machine learning is a powerful tool that can help solve a wide range of problems. With Python, you have access to a vast ecosystem of libraries and tools that make implementing ML models straightforward. Start with simple models, experiment, and gradually move towards more complex projects. Happy coding!
Would you like to add more details on any specific section or explore advanced topics in machine learning?